Examples
These example codes can be found in /usr/share/doc/murange/examples/.
Table of Contents
- Example 1: Calculate the range of a 200 MeV/c muon in Iron
- Example 2: Print a stopping power and range table for Yttium (Y)
- Example 3: Calculate energy loss iteratively to estimate the computing performance
- Example 4: Calculate energy range of a 25 GeV muon in Carbon Tetrafluoride at 1 atm and 4 Torr
Example 1
The following example code creates an element, a range table for the element, a muon object with momentum 200 MeV/c and outputs the range,
#include <iostream>
#include <murange.hpp>
int main() {
// Iron (Fe)
Element *Fe = new Element("Fe");
// range table for element Fe
Range *rFe = new Range(Fe);
// muon with momentum 200 MeV/c
Muon *mu = new Muon();
mu->SetMomentum(200);
cout << rFe->GetRange(mu) << " MeV/cm2" << endl;
delete Fe;
delte rFe;
delete mu;
return EXIT_SUCCESS;
}
The outcome of this example is,
$ ./fe-range
56.7843 MeV/cm2
Example 2
The following example code creates an element (Yttrium) and prints to standard output a stopping power and range table,
#include <iostream>
#include <murange.hpp>
int main() {
// Yttrium (Y)
Element *Y = new Element("Y");
// print stopping power and range table
Y->PrintRangeTable();
delete Y;
return EXIT_SUCCESS;
}
Example 3
The following example code creates a muon object, an element (Si), a range table and sets the muon kinetic energy randomly, from 10 to 100 MeV, then calculates the energy loss in 1-10 g/cm2 Si, iterated over tries times. The OpenMP time function is used to estimate the performance [Mtrials/s],
#include <iostream>
#include <ctime>
#include <murange.hpp>
#include <omp.h>
int main(int argc, char **argv) {
// number of tries
unsigned long tries = atol(argv[1]);
srand(time(NULL));
// start time
double start_time = omp_get_wtime();
// muon, Si, range table
Muon *mu = new Muon();
Element *Si = new Element("Si");
Range *rSi = new Range(Si);
for ( int i = 0 ; i < tries ; i++ ) {
// kinetic energy between 10 and 100 MeV
double ke = (rand()%(90000000)+10000000)/1000000.;
mu->SetKEnergy(ke);
// thickness between 1 and 10 g/cm2
double s = (rand()%(90000000)+10000000)/10000000.;
rSi->GetOutgoingEnergy(mu,s);
}
// stop time
double stop_time = omp_get_wtime();
double performance = (double)tries / (time_stop-time_start) / 1000000.;
fprintf(stderr,"\nnumber of tries: %ld\n",tries);
fprintf(stderr,"execution time: %g s\n",time_stop-time_start);
fprintf(stderr,"performance: %g Mtrials/s\n\n",performance);
delete mu;
delete Si;
delete rSi;
return EXIT_SUCCESS;;
}
The outcome of this example is,
$ ./performance 100000000
number of tries: 100000000
execution time: 18.8887 s
performance: 5.29418 Mtrials/s
The plot shows the performance as a function of number of trials, a useful measure when performing Monte Carlo simulations.
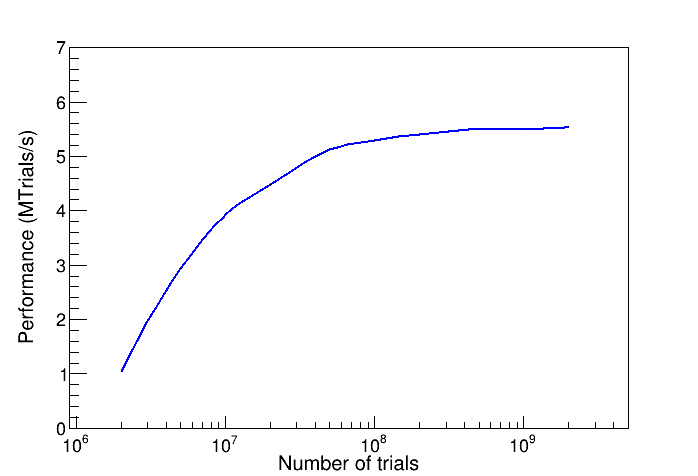
A similar OpenMP version of the above code, with 8 threads gives,
$ ./performance-omp 8 100000000
using OpenMP with 8/16 threads
number of tries: 100000000
execution time: 3.22482 s
performance: 31.0095 Mtrials/s
and performance plot,
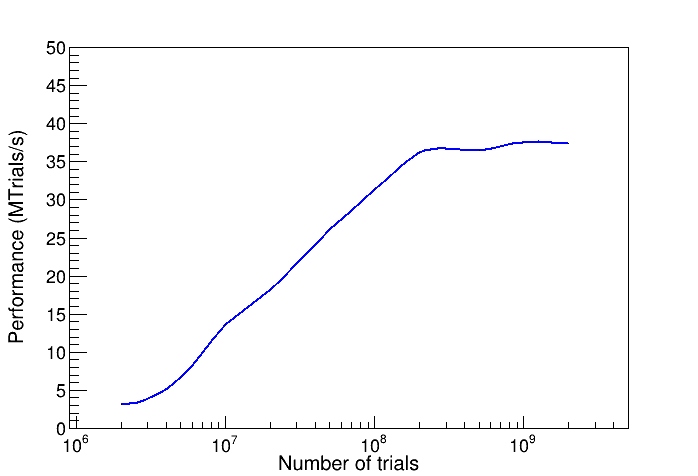
Example 4
The following example code calculates the range of a 25 GeV muon in Carbon Tetrafluoride (CF4) at 1 atm and 4 Torr,
#include <iostream>
#include <murange.hpp>
int main() {
// range table for CF4
Compound *CF4 = new Compound(326);
Range *rCF4 = new Range(CF4);
// muon with kinetic energy 25 GeV
Muon *mu = new Muon(25e3);
cout << "Range of" << mu->GetKEnergy()/1000 << " GeV muon in CF4 at 1 atm: " << rCF4->GetRange(mu) << " MeV/cm2" << endl;
// change the density to 4 Torr
CF4->SetDensity(1.8942e-5);
rCF4 = new Range(CF4);
cout << "Range of" << mu->GetKEnergy()/1000 << " GeV muon in CF4 at 4 Torr: " << rCF4->GetRange(mu) << " MeV/cm2" << endl;
delete CF4;
delte rCF4;
delete mu;
return EXIT_SUCCESS;
}
The outcome of this example is,
$ ./cf4-range
Range of 25 GeV muon in CF4 at 1 atm: 10308.6 MeV/cm2
Range of 25 GeV muon in CF4 at 4 Torr: 10107.5 MeV/cm2
This demostrates the density dependence of the electronic stopping power.
← Return to Muon Range Library